A lot of web products under test require some level of authentication. When QA engineers test such software, they have to be able to operate a particular testing user. As a rule, it’s generated randomly and then is used in most tests. Further, in the article, we’ll talk about the most popular types of user generation in Cypress tests.
This material is of a technical nature. Therefore, it is understandable to those who are experienced in this product (for example, those who perform e-commerce testing).
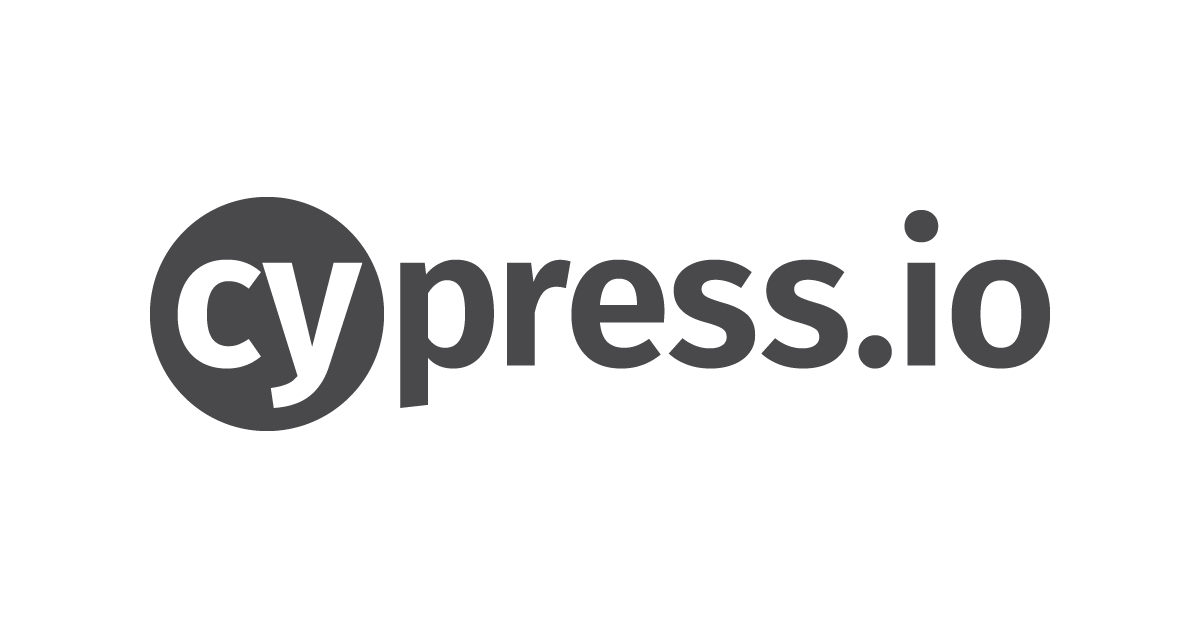
cypress.io
Working with Hooks
We can take a support/index.js file and put before() or beforeEach() hook there.
As a result, we’ll get the following:
const { internet } = require(‘faker’);
const email = internet.exampleEmail()
const password = internet.password()
beforeEach(() => {
cy
.request(‘POST’, ‘/signup’, { email, password })
.then(({ body }) => {
cy
.setCookie(‘trello_token’, body.accessToken);
});
});
Faker, an auxiliary tool, allows generating different parameters of testing emails for each user test.
The authorization process can create a lot of test data before each test. On the one hand, this procedure allows tests to be independent of each other. And that’s great! But creating a new user for each test is a hard and time-consuming process.
Creating a Test Script
Usually, before starting the test through Cypress run, it’s possible to run scripts that automatically create a user and then save it to a particular file. After, QA specialists use this file during testing to authorize a virtual user.
The content of testing signup.js:
const axios = require(‘axios’)
const { internet } = require(‘faker’);
const email = internet.exampleEmail()
const password = internet.password()
const fs = require(‘fs’)
const signupUser = async () => {
const user = await axios
.post(‘http://localhost:3000/signup’, { email, password })
fs.writeFileSync(“./cypress/data.json”, JSON.stringify(user.data))
}
signupUser()
You can run it as a separate test script that is defined in the package.json file.
package.json
“scripts”: {
“start”: “cd trelloapp && npm start”,
“cy:run”: “cypress run”,
“createUser”: “node signupUser.js”
}
Also, you can run this script through npm run createUser, and then run the following tests: npm run cy:run.
cypress/plugins/index.js
const signupUser = require(‘../../signupUser.js’)
module.exports = async (on, config) => {
config.env = await signupUser()
return config;
}
Cypress will have to wait till the signupUser() function is executed, and then save the data that was returned by the parameter to the config object. In that case, the information is generated during test performance and will be available only when Cypress runs. If a user runs tests in parallel (for example, on 15 local machines), then this script will be called 15 times. Perhaps, it’s for the best, as in that case, users won’t interfere with each other.
Leave A Comment